Creating scatter plots
Note: Where possible, use qlik-embed and qlik-api rather than this framework.
This section describes how to create scatter plots with the Visualization API.
Basic scatter plot with number formatting
In this example, you will create a basic scatter plot, containing one dimension and two measures. The measures have basic number formatting applied and a title is added to the scatter plot.
-
Create the chart.
Create the container for the chart. The visualization type is
scatterplot
.app.visualization.create('scatterplot',[],{}) -
Define the dimension.
Define the dimension as a column.
{"qDef": {"qFieldDefs": ["=[Date.autoCalendar.YearQuarter]"],"qFieldLabels": ["Year & Qtr"]},"qNullSuppression": true} -
Define the first measure.
Define the first measure as a column.
{"qDef": {"qFieldDefs": ["=[Date.autoCalendar.YearQuarter]"],"qFieldLabels": ["Year & Qtr"]},"qNullSuppression": true},{"qDef": {"qLabel": "FIR%","qDef": "Avg(FwHit)","qNumFormat": {"qType": "F","qnDec": 2,"qUseThou": 0,"qFmt": "0.0%","qDec": ".","qThou": ","}}} -
Define the second measure.
Define the second measure as a column.
{"qDef": {"qFieldDefs": ["=[Date.autoCalendar.YearQuarter]"],"qFieldLabels": ["Year & Qtr"]},"qNullSuppression": true},{"qDef": {"qLabel": "FIR%","qDef": "Avg(FwHit)","qNumFormat": {"qType": "F","qnDec": 2,"qUseThou": 0,"qFmt": "0.0%","qDec": ".","qThou": ","}}},{"qDef": {"qLabel": "GIR%","qDef": "Avg(GIR)","qNumFormat": {"qType": "F","qnDec": 2,"qUseThou": 0,"qFmt": "0.0%","qDec": ".","qThou": ","}}} -
Define the title.
Then define the title in the options.
{"showTitles": true,"title": "Fairways hit vs. Greens hit relation"}
Result
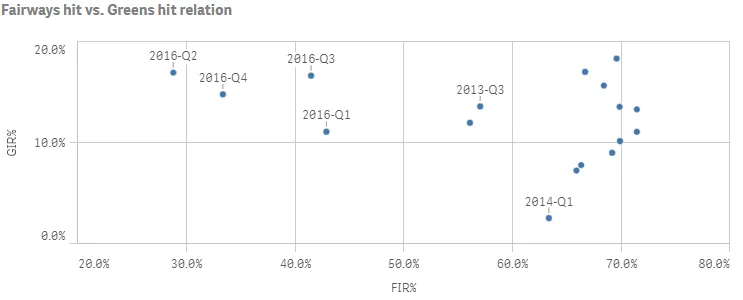
Complete code example: Basic scatter plot chart
-
Visualization API
const config = {host: '<TENANT_URL>', //for example, 'abc.us.example.com'prefix: '/',port: 443,isSecure: true,webIntegrationId: '<WEB_INTEGRATION_ID>'};require.config({baseUrl: `https://${config.host}/resources`,webIntegrationId: config.webIntegrationId});require(["js/qlik"], (qlik) => {qlik.on('error', (error) => console.error(error));const app = qlik.openApp('<APP_ID>', config);app.visualization.create('scatterplot',[{"qDef": {"qFieldDefs": ["=[Date.autoCalendar.YearQuarter]"],"qFieldLabels": ["Year and Qtr"]},"qNullSuppression": true},{"qDef": {"qLabel": "FIR%","qDef": "Avg(FwHit)","qNumFormat": {"qType": "F","qnDec": 2,"qUseThou": 0,"qFmt": "0.0%","qDec": ".","qThou": ","}}},{"qDef": {"qLabel": "GIR%","qDef": "Avg(GIR)","qNumFormat": {"qType": "F","qnDec": 2,"qUseThou": 0,"qFmt": "0.0%","qDec": ".","qThou": ","}}}],{"showTitles": true,"title": "Fairways hit vs. Greens hit relation"}).then((vis)=>{vis.show("QV01");});});
Using calculated bubble size
In this example, you will add a third measure to the scatter plot, which is used to calculate the bubble size.
-
Define the third measure.
Define the third measure as a column.
{"qDef": {"qLabel": "Holes played","qDef": "Count(HoID)"}} -
Define the bubble size range.
The bubble size range is defined in the
dataPoint
object in the options:"dataPoint": { "rangeBubbleSizes": [ 1, 11 ] }
.Note:
rangeBubbleSizes
is an array of integers where the first index is the from-size, and the second is the to-size. From-size should always be smaller than the to-size. 1 is the minimum value and 20 is the maximum value.{"showTitles": true,"title": "Fairways hit vs. Greens hit relation","dataPoint": {"rangeBubbleSizes": [1,11]}}
Result
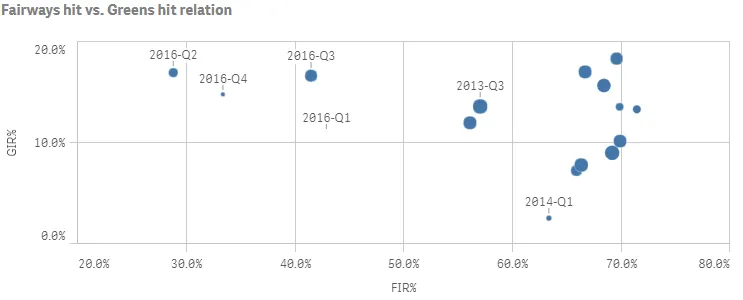
Complete code example: Scatter plot chart with bubble size modified by data
-
Visualization API
const config = {host: '<TENANT_URL>', //for example, 'abc.us.example.com'prefix: '/',port: 443,isSecure: true,webIntegrationId: '<WEB_INTEGRATION_ID>'};require.config({baseUrl: `https://${config.host}/resources`,webIntegrationId: config.webIntegrationId});require(["js/qlik"], (qlik) => {qlik.on('error', (error) => console.error(error));const app = qlik.openApp('<APP_ID>', config);app.visualization.create('scatterplot',[{"qDef": {"qFieldDefs": ["=[Date.autoCalendar.YearQuarter]"],"qFieldLabels": ["Year and Qtr"]},"qNullSuppression": true},{"qDef": {"qLabel": "FIR%","qDef": "Avg(FwHit)","qNumFormat": {"qType": "F","qnDec": 2,"qUseThou": 0,"qFmt": "0.0%","qDec": ".","qThou": ","}}},{"qDef": {"qLabel": "GIR%","qDef": "Avg(GIR)","qNumFormat": {"qType": "F","qnDec": 2,"qUseThou": 0,"qFmt": "0.0%","qDec": ".","qThou": ","}}},{"qDef": {"qLabel": "Holes played","qDef": "Count(HoID)"}}],{"showTitles": true,"title": "Fairways hit vs. Greens hit relation","dataPoint": {"rangeBubbleSizes": [1,11]}}).then((vis)=>{vis.show("QV01");});});
Customizing the bubble colors
In this example, you will customize the color of the bubbles by an expression, where three different colors are applied depending on the return value of the expression.
-
Enable custom colors.
The bubble color is defined in the color object. You will enable custom coloring
"auto": false
and set"mode": "byExpression"
. Then define the expression to be used for coloring:"colorExpression": "If(Avg(Stableford)>2,rgb(82, 162, 204),
If(Avg(Stableford)<1.65,rgb(248, 152, 29),rgb(128, 128, 128)))"
. Since the expression includes an actual color code, set"expressionIsColor": true
."color": {"auto": false,"mode": "byExpression"} -
Define the color expression.
You then define the expression to be used for coloring:
"colorExpression":
"If(Avg(Stableford)>2,rgb(82, 162, 204),If(Avg(Stableford)<1.65,rgb(248,
152, 29),rgb(128, 128, 128)))"
. Since the expression includes an actual color code,"expressionIsColor": true
."color": {"auto": false,"mode": "byExpression","expressionIsColor": true,"colorExpression": "If(Avg(Stableford)>2,rgb(82, 162, 204),If(Avg(Stableford)<1.65,rgb(248, 152, 29),rgb(128, 128, 128)))"} -
Add an attribute expression on the first measure.
Since color expressions must be added as attribute expressions on the first measure, you define this inside the first measure definition in the columns:
"qAttributeExpressions": [ { "qExpression": "If(Avg(Stableford)>2,rgb(82,
162, 204), If(Avg(Stableford)<1.65,rgb(248, 152, 29),rgb(128, 128,
128)))", "id": "colorByExpression" } ]
.{"qDef": {"qLabel": "FIR%","qDef": "Avg(FwHit)","qNumFormat": {"qType": "F","qnDec": 2,"qUseThou": 0,"qFmt": "0.0%","qDec": ".","qThou": ","}},"qAttributeExpressions": [{"qExpression": "If(Avg(Stableford)>2,rgb(82, 162, 204),If(Avg(Stableford)<1.65,rgb(248, 152, 29),rgb(128, 128, 128)))","id": "colorByExpression"}]},
Result
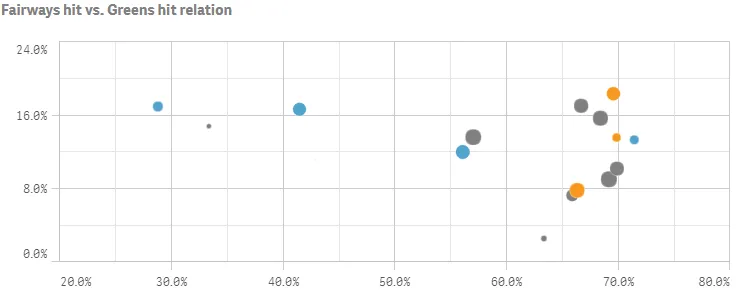
Complete code example: Scatter plot chart with colour and attribute modifiers
-
Visualization API
const config = {host: '<TENANT_URL>', //for example, 'abc.us.example.com'prefix: '/',port: 443,isSecure: true,webIntegrationId: '<WEB_INTEGRATION_ID>'};require.config({baseUrl: `https://${config.host}/resources`,webIntegrationId: config.webIntegrationId});require(["js/qlik"], (qlik) => {qlik.on('error', (error) => console.error(error));const app = qlik.openApp('<APP_ID>', config);app.visualization.create('scatterplot',[{"qDef": {"qFieldDefs": ["=[Date.autoCalendar.YearQuarter]"]},"qNullSuppression": true},{"qDef": {"qLabel": "FIR%","qDef": "Avg(FwHit)","qNumFormat": {"qType": "F","qnDec": 2,"qUseThou": 0,"qFmt": "0.0%","qDec": ".","qThou": ","}},"qAttributeExpressions": [{"qExpression": "If(Avg(Stableford)>2,rgb(82, 162, 204), If(Avg(Stableford)<1.65,rgb(248, 152, 29),rgb(128, 128, 128)))","id": "colorByExpression"}]},{"qDef": {"qLabel": "GIR%","qDef": "Avg(GIR)","qNumFormat": {"qType": "F","qnDec": 2,"qUseThou": 0,"qFmt": "0.0%","qDec": ".","qThou": ","}}},{"qDef": {"qLabel": "Holes played","qDef": "Count(HoID)"}}],{"showTitles": true,"title": "Fairways hit vs. Greens hit relation","showDetails": false,"navigation": false,"dataPoint": {"bubbleSizes": 5,"rangeBubbleSizes": [1,11]},"labels": {"mode": 0},"compressionResolution": 5,"gridLine": {"auto": false,"spacing": 3},"color": {"auto": false,"mode": "byExpression","useBaseColors": "off","expressionIsColor": true,"colorExpression": "If(Avg(Stableford)>2,rgb(82, 162, 204),If(Avg(Stableford)<1.65,rgb(248, 152, 29),rgb(128, 128, 128)))"},"xAxis": {"show": "labels"},"yAxis": {"show": "labels"}}).then((vis)=>{vis.show("QV01");});});