Create Signed Tokens for JWT Authorization
JWT solutions leverage cookies for auth, which are blocked by some browser vendors. If you can’t use OAuth2 impersonation in your solution, you may need to use a JWT proxy.
Overview
In this tutorial, you are going to learn how to configure Qlik Cloud to use JSON web tokens (JWT) for authorizing users in your tenant.
Contents:
- Before you begin
- Create a public / private key pair for signing JWTs
- Configure JWT identity provider
- JWT format for Qlik Sense authorization
- Example JWT signing code
Before you begin
- Only a user with the
Tenant Admin
role may access the management console settings and add roles to users. If you aren’t theTenant Admin
of your Qlik Sense SaaS tenant, contact yourTenant Admin
and direct them to this tutorial.
Create a public / private key pair for signing JWTs
Open a terminal window and use OpenSSL to generate public and private key. Store the private key someplace safe and don’t share it. The public key is used in the configuration section.
openssl genrsa -out privatekey.pem 4096
openssl req -new -x509 -key privatekey.pem -out publickey.cer -days 1825
If you are using Windows, you need to download and install OpenSSL. You can obtain a copy of OpenSSL from the Win32/Win64 OpenSSL Installation Project page.
Configure JWT identity provider
Inside the management console, click the Identity provider menu item on the left side of the screen.
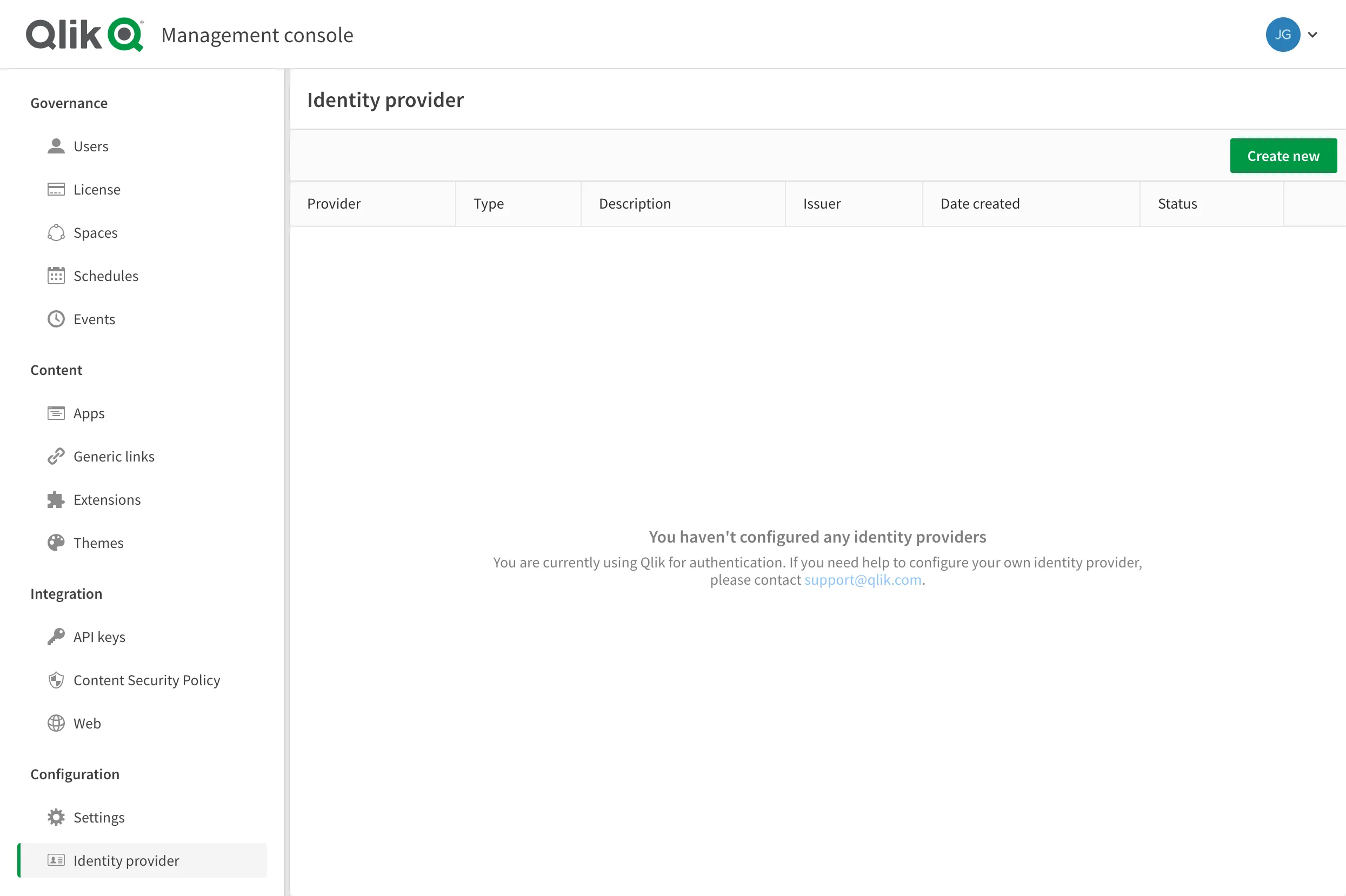
Click the Create new button to begin the configuration. When the configuration panel appears, click the Type dropdown control and choose JWT from the list.
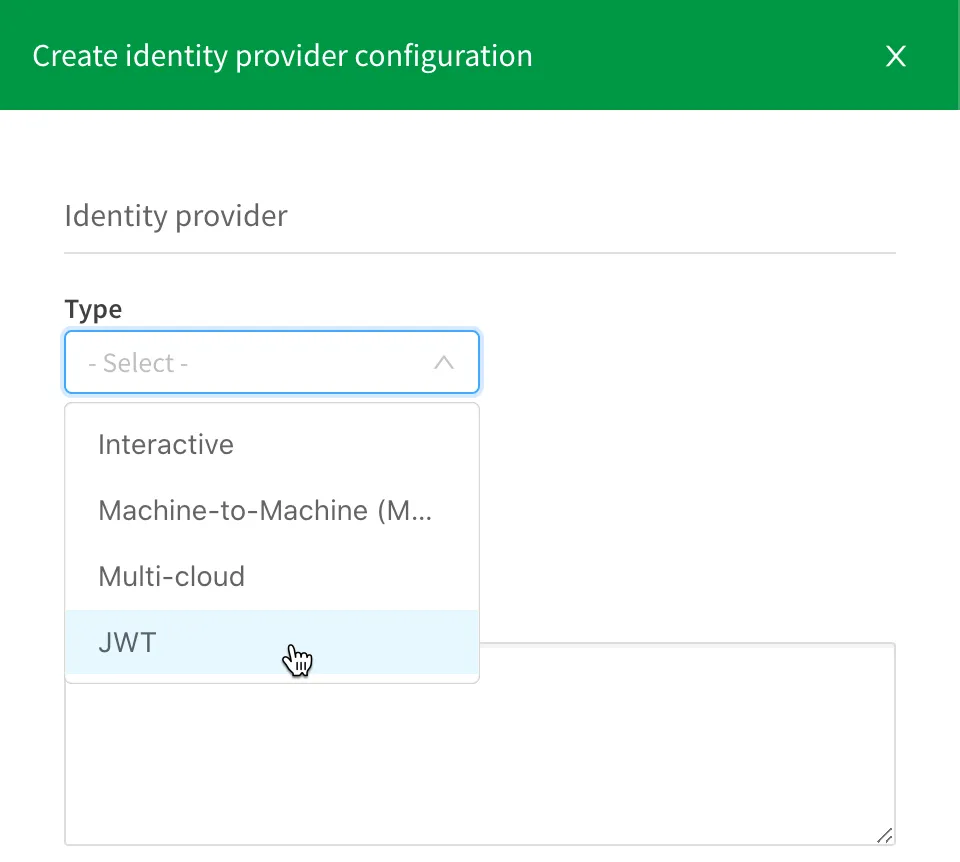
The remaining configuration dialogs appear after making the selection from the dropdown. There are four input boxes to fill in:
- Description
- Certificate (required) - the placeholder for the public key created earlier.
- Issuer (required) - the value input here identifies the application or service that issues the JWT.
- Key ID (required) - the value input here informs the application which signing key to use.
If an Issuer or Key ID isn’t supplied during configuration, values automatically generate upon creation. These claims need to be supplied in the JWT for Qlik to trust the JWT and authorize users.
Add the public key to the configuration
Open the file containing the public key in your favorite text editor.
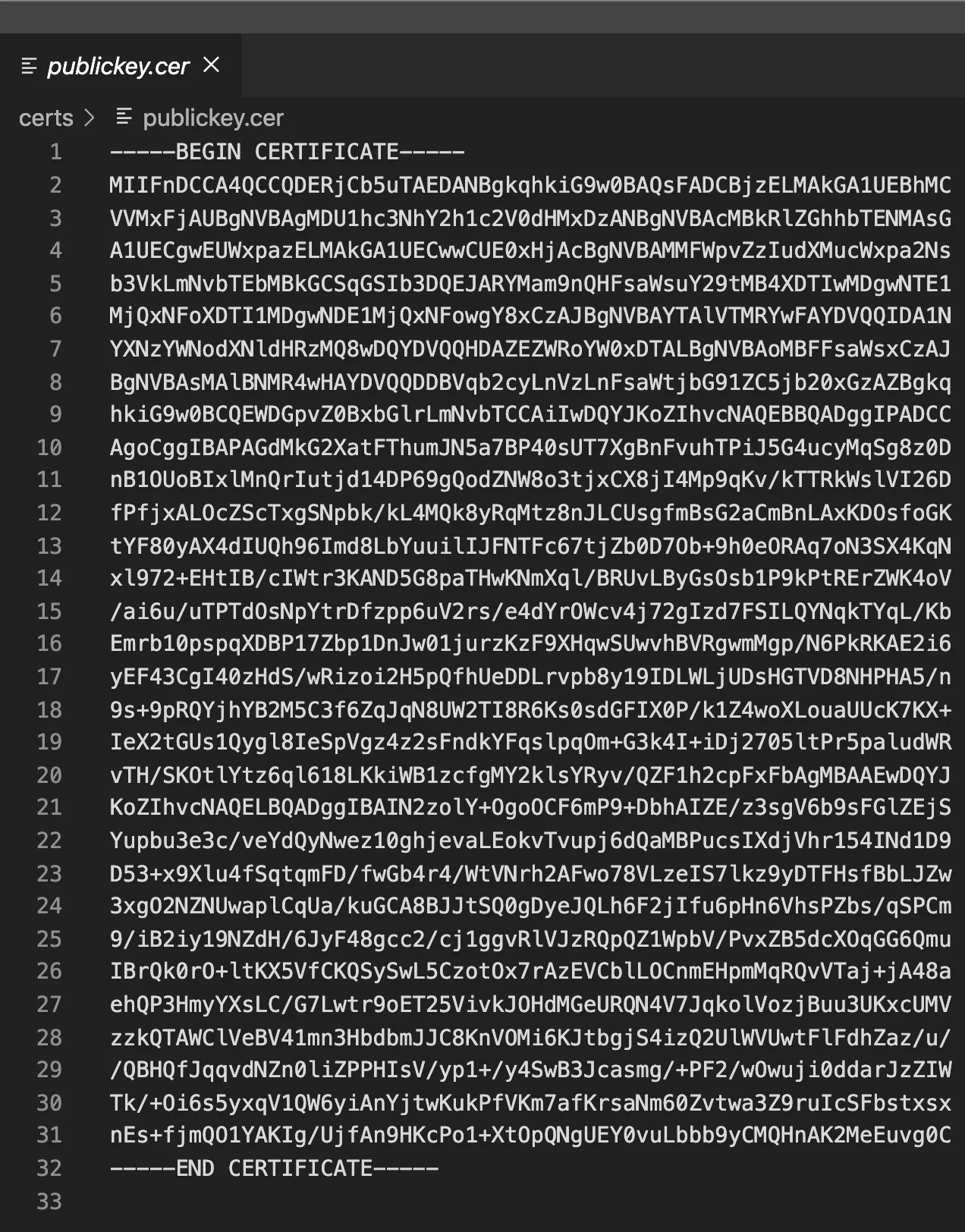
Remove any carriage returns or line feeds existing in the text. In Visual Studio
Code, removing the characters can be done using a regular expression find and
replace on \n
. Once the characters are removed, the text should appear in the
editor as a single line with no spaces.
Copy the text and paste it into the certificate input field in the configuration.
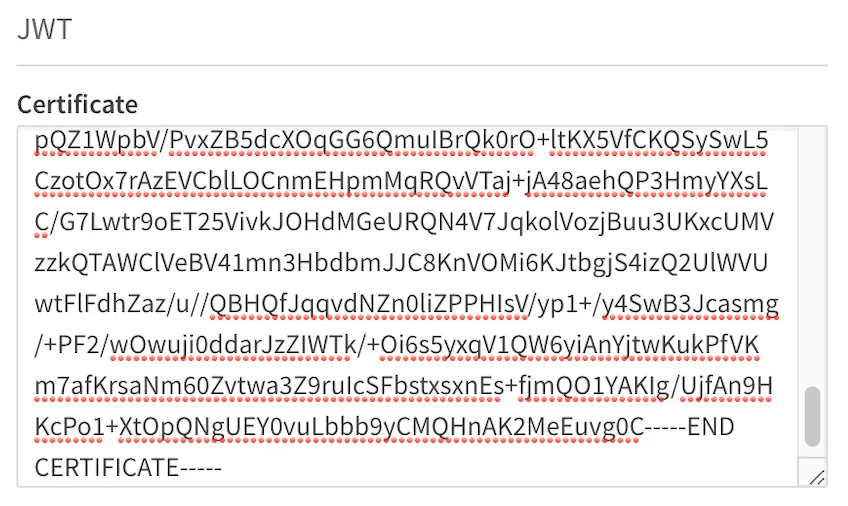
A public key certificate with trailing spaces, carriage returns, or line feeds triggers an error upon saving the configuration.
Input Issuer and Key ID values
As stated previously, adding an Issuer or Key ID is optional. However, they’re required attributes (claims) in the JWT sent to Qlik. Both values are strings so entering any information here is suitable as long as a matching value exists in the JWT.
When no Issuer or Key ID values are supplied, upon completing the configuration the management console presents a window with generated values.
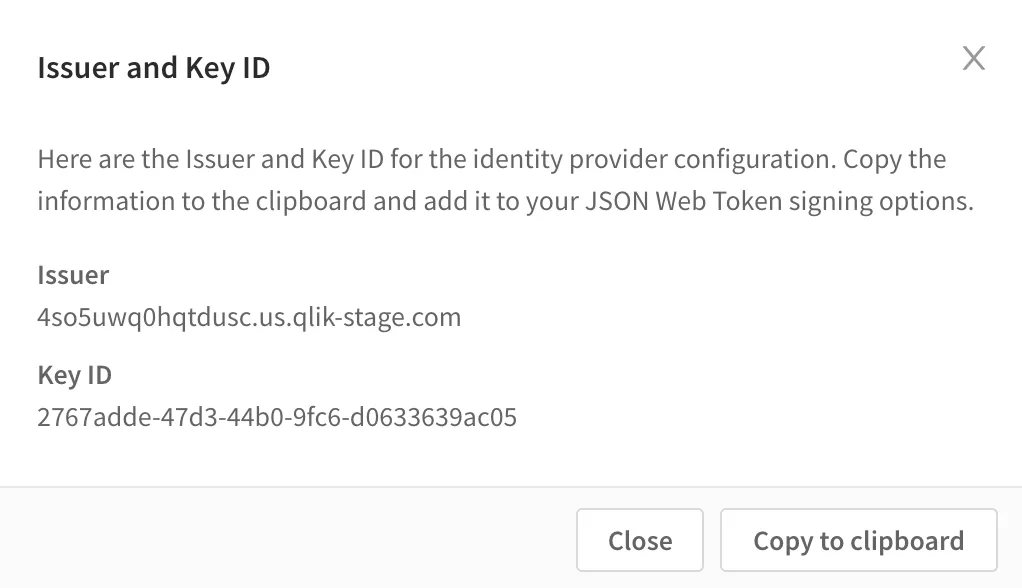
Alternatively, entering Issuer and Key ID values surface the input values in the confirmation screen.
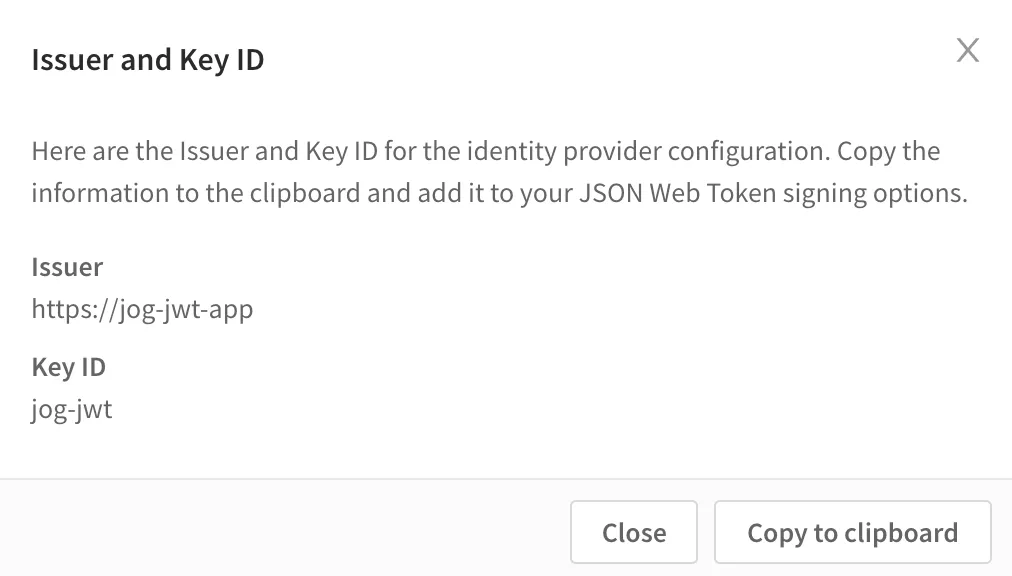
Complete the configuration
Press the Create button to save the JWT authorization configuration. The confirmation screen appears to copy the Issuer and Key ID values to the clipboard.
JWT format for Qlik Sense authorization
The JWT is made of three pieces:
- The payload.
- The signing options.
- The private key for signing the token.
The payload
The required claims for a Qlik JWT payload are the following:
- jti - (JWT ID) claim provides a unique identifier for the JWT.
- iat - The issued at date of the JWT provided as a Unix epoch timestamp.
- sub - The main identifier (aka subject) of the user.
- subType - The type of identifier the sub represents. In this case,
user
is the only applicable value. - name - The friendly name to apply to the user.
- email - The email address of the user.
- email_verified - A claim indicating to Qlik that the JWT source has verified that the email address belongs to the subject.
- iss - This is a value created or supplied previously with identity provider configuration. It can be a random string.
- exp - The date/time the JWT expires. The time difference between
nbf
andexp
cannot exceed 1 hour (3600 seconds). - nbf - the date/time before which the JWT MUST NOT be accepted for processing.
The time difference between
nbf
andexp
cannot exceed 1 hour (3600 seconds). - aud - A required value instructing the Qlik platform how to treat the received JWT.
It’s possible to send additional claims in the JWT. Today,
only the optional claim groups
is read.
Here is a sample JWT payload including the groups attribute:
{
"jti": "k5bU_cFI4_-vFfpJ3DjDsIZK-ZhJGRbBfusUWZ0ifBI",
"iat": 1658416389,
"sub": "SomeSampleSeedValue", //e.g. 0hEhiPyhMBdtOCv2UZKoLo4G24p-7R6eeGdZUQHF0-c
"subType": "user",
"name": "Hardcore Harry",
"email": "harry@example.com",
"email_verified": true,
"groups": ["Administrators", "Sales", "Marketing"]
}
Depending on the library you use to sign JWTs, you may have to manually assign
an iat
value to the JWT payload. Many JWT signing libraries -
like JSONWebToken for Node - add the iat
attribute automatically upon JWT
signing. Refer to the documentation for the JWT signing library you are
using to determine iat
handling.
The signing options
The required properties for the signing options of the JWT are as follows:
- kid - This is a value created or supplied previously with identity provider configuration. It can be a random string.
- alg - The encryption algorithm to protect the JWT with the private key.
Here is a sample of JWT signing options:
{
"kid": "my-custom-jwt",
"alg": "RS256",
"iss": "https://my-custom-jwt",
"exp": "5m", //Expires 5 minutes after the issue date/time.
"nbf": "1s", //JWT is valid 1 second after the issue date/time.
"aud": "qlik.api/login/jwt-session"
}
The time between nbf
and exp
must not exceed sixty minutes.
Combining the payload, the signing options, and the private key using a library like jsonwebtoken in JavaScript produces a token.
Example of JWT signing code
The following code is a sample of an express application that creates JWTs.
const fs = require('fs');
const uid = require('uid-safe');
const jwt = require('jsonwebtoken');
const payload = {
jti: uid.sync(32), // 32 bytes random string
sub: '0hEhiPyhMBdtOCv2UZKoLo4G24p-7R6eeGdZUQHF0-c',
subType: 'user',
name: 'Hardcore Harry',
email: 'harry@example.com',
email_verified: true,
groups: ['Administrators', 'Sales', 'Marketing'],
};
const privateKey = fs.readFileSync('./certs/privatekey.pem');
// kid and issuer have to match with the IDP config and the
// audience has to be qlik.api/jwt-login-session
const signingOptions = {
kid: 'my-custom-jwt',
alg: 'RS256',
iss: 'https://my-custom-jwt',
exp: '5m',
nbf: '1s',
aud: 'qlik.api/login/jwt-session',
};
const myToken = jwt.sign(payload, privateKey, signingOptions);
console.log(myToken);
Get started with Catalog integration
Learn the basics of using the Catalog API.
Get started with Lineage integration
Learn the basics using the lineage API.
Build a web app for the Insight Advisor API
Learn how to access the Insight Advisor API using a web app.