Create bookmarks (Platform SDK)
qlik/api: The typescript platform SDK used in this tutorial has been deprecated. Review how to transition to qlik/api.
Introduction
In this tutorial, you are going to learn how to create a bookmark with the Platform SDK.
Requirements
- A Qlik Cloud tenant
- An API key or machine-to-machine OAuth client to authenticate to your tenant
- Executive Dashboard Qlik Sense App imported into your tenant
- Typescript Platform SDK
- NodeJs version 18 or higher
- Basic knowledge of JavaScript
Variables you need to complete this tutorial
- host: The hostname for your Qlik Cloud tenant
(for example
hello.us.qlikcloud.com
) - clientId: The
clientId
for the machine-to-machine OAuth client - clientSecret: The
clientSecret
for the machine-to-machine OAuth client - appId The
appId
is the unique identifier (guid) for the application you want to connect to.
Authorize a connection to Qlik Cloud
Configure a Nodejs application and add the @qlik/sdk
package using NPM.
npm install @qlik/sdk
Create a file named bookmark-create.js
file of your application you want to
use the SDK, import the Qlik
and AuthType
modules from the SDK.
const Qlik = require('@qlik/sdk').default;
const { AuthType } = require("@qlik/sdk");
Update the configuration values with the variables identified earlier in the tutorial.
const host = "<tenant.region.qlikcloud.com>";
const clientId = "<OAUTH_CLIENT_ID>";
const clientSecret = "<OAUTH_CLIENT_SECRET>";
const appId = "<APPID_GUID_LIKE_THIS_b1b79fcd-e500-491c-b6e9-2ceaa109214c";
Configure authorization to Qlik. This example uses a machine-to-machine OAuth token to connect to Qlik Cloud.
const config = {
authType: AuthType.OAuth2,
host: host,
clientId: clientId,
clientSecret: clientSecret
};
Create a bookmark
Begin with an async
function to immediately execute this snippet. The
remainder of the code goes in between the curly {}
braces.
(async () => {})();
Establish a connection to your Qlik Cloud tenant.
const qlik = new Qlik(config);
await qlik.auth.authorize();
Get a reference to the Qlik Sense application to open and open it.
const app = await qlik.apps.get(appId);
await app.open();
Create the bookmark using the createBookmarkEx
function with a JSON payload
containing the following structure and values.
const bmk = await app.createBookmarkEx(
{
"creationDate": new Date().toISOString(),
"qInfo": {
"qType": "bookmark",
},
"qMetaDef": {
"qName": "hello-bookmark",
"title": "hello-bookmark",
"description": "Hello! This is a bookmark created with a snippet from qlik.dev.",
"isExtended": true
}
});
creationDate
: The date the bookmark is being created in ISO format.qType
: The type of object being created. In this case, abookmark
. Must be part of theqInfo
object.qName
: The internal name for the bookmark. Must be part of theqMetaDef
object.title
: The title is the value shown in the Qlik Sense user interface when users access bookmarks. Must be part of theqMetaDef
object.description
: A snippet of text for telling users what the bookmark is going to do. Must be part of theqMetaDef
object.isExtended
: Who knows what this is for?
When the bookmark is created, you can review the bookmark reference using the
getLayout()
function.
const bmkLayout = await bmk.getLayout();
console.log(bmkLayout);
To make the bookmark available to all users in the app, you need to publish the
bookmark for sharing. You can do this by calling the publish
function.
const pubBmk = await bmk.publish();
Run the script by issuing the command node create-bookmark.js
. The response
returned in the console will be the layout information describing the bookmark.
GenericBookmarkLayout {
qBookmark: NxBookmark {
qPatches: [],
qStateData: [ [AlternateStateData] ],
qUtcModifyTime: 45023.02179398148,
qVariableItems: []
},
qFieldInfos: [],
qInfo: NxInfo {
qId: 'b5be3c5c-7d03-48fb-9ca8-8eb926a26c90',
qType: 'bookmark'
},
qMeta: NxMeta {
qName: 'hello-bookmark',
privileges: [ 'read', 'update', 'delete', 'publish' ],
title: 'hello-bookmark',
description: 'Hello! This is a bookmark created with a snippet from qlik.dev.',
isExtended: true
},
creationDate: '2023-04-07T00:31:23.847Z'
}
Open the Qlik Sense application in a browser and review the public bookmarks. The bookmark you created will appear in the list.
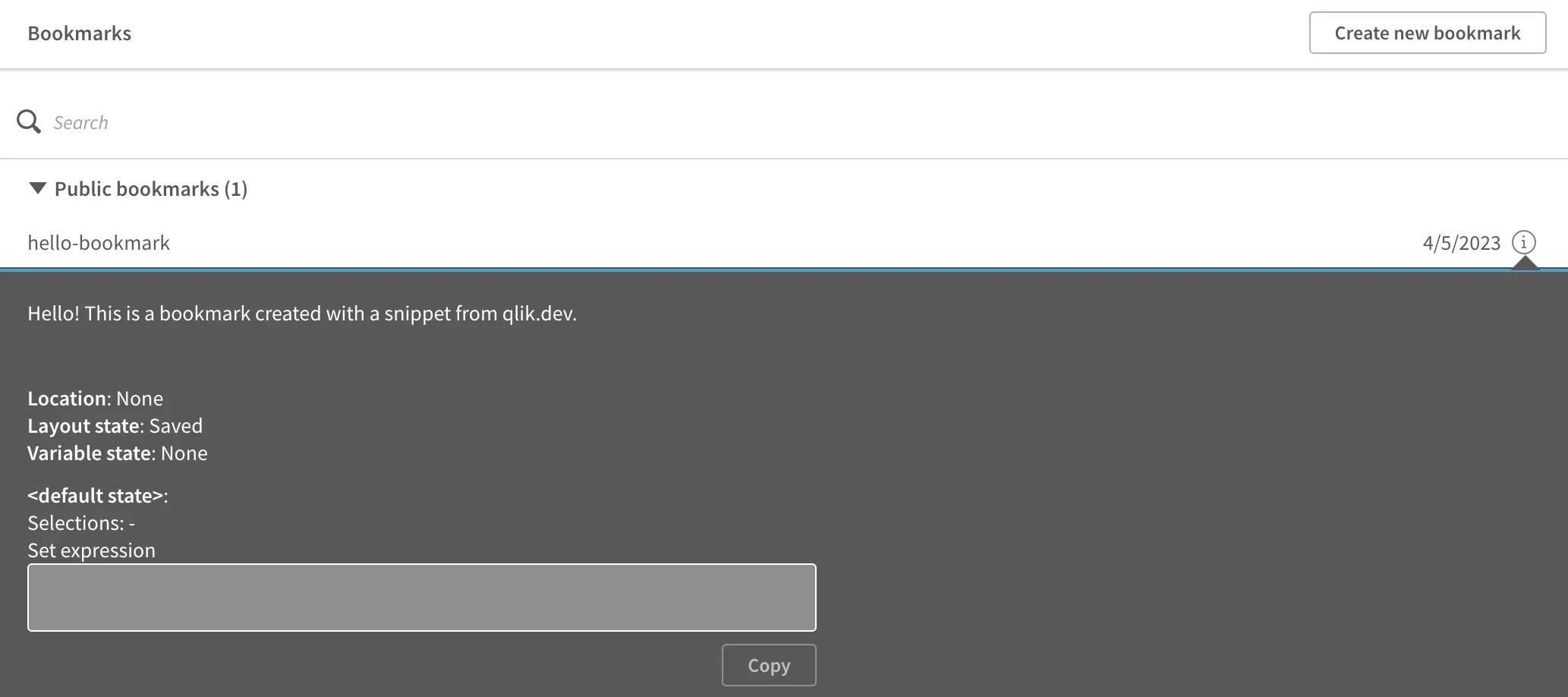
Next steps
Now that you’ve created a bookmark - or multiple bookmarks - you want to be able to list them out. Check out this tutorial on accessing bookmark lists from code.
Full code snippet
//create-bookmark.js
//Create and publish a bookmark
//Authorizes through OAuth2 M2M to connect to a Qlik Cloud application.
//To create an OAuth client for this snippet, go here:
//https://qlik.dev/authenticate/oauth/create/create-oauth-client
//To use a sample app with this snippet, download and import the executive
//dashboard from this github location https://github.com/qlik-oss/qlik-cloud-examples/raw/main/qlik.dev/sample-apps/qlik-dev-exec-dashboard.qvf
/*PARAMS
* host: the hostname of your tenant
* clientId: the clientId of the OAuth2 client you created
* clientSecret: the client secret for the OAuth2 client you created
* appId: The GUID for the Qlik Sense app
*/
//Uncomment below if you're using this code with https://repl.it
//global.fetch = require('@replit/node-fetch');
const Qlik = require('@qlik/sdk').default;
const { AuthType } = require("@qlik/sdk");
//config-values
const host = "<tenant.region.qlikcloud.com>";
const clientId = "<OAUTH_CLIENT_ID>";
const clientSecret = "<OAUTH_CLIENT_SECRET>";
const appId = "<APPID_GUID_LIKE_THIS_b1b79fcd-e500-491c-b6e9-2ceaa109214c";
const config = {
authType: AuthType.OAuth2,
host: host,
clientId: clientId,
clientSecret: clientSecret
};
(async () => {
const qlik = new Qlik(config);
await qlik.auth.authorize();
const app = await qlik.apps.get(appId);
await app.open();
const bmk = await app.createBookmarkEx(
{
"creationDate": new Date().toISOString(),
"qInfo": {
"qType": "bookmark",
},
"qMetaDef": {
"qName": "hello-bookmark",
"title": "hello-bookmark",
"description": "Hello! This is a bookmark created with a snippet from qlik.dev.",
"isExtended": true
}
});
const bmkLayout = await bmk.getLayout();
console.log(bmkLayout);
const pubBmk = await bmk.publish();
console.log(pubBmk);
process.exit();
})();